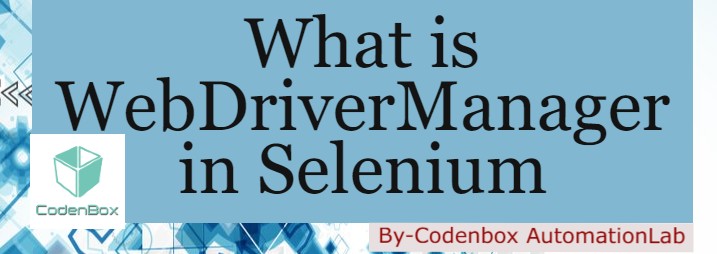
As an automation engineer we know that we need to use setProperty() method to set and let our WebDriver know the key and the path of our browser binary (executable file) due to lunch or handle some browsers such as Chrome, Firefox, Opera, PhantomJS, Microsoft Edge, or Internet Explorer. First we need to download the binary file and must be set as JVM properties as follows:
System.setProperty(“webdriver.chrome.driver”, “/path/to/binary/chromedriver”);
System.setProperty(“webdriver.gecko.driver”, “/path/to/binary/geckodriver”);
System.setProperty(“webdriver.opera.driver”, “/path/to/binary/operadriver”);
System.setProperty(“phantomjs.binary.path”, “/path/to/binary/phantomjs”);
System.setProperty(“webdriver.edge.driver”, “C:/path/to/binary/msedgedriver.exe”);
System.setProperty(“webdriver.ie.driver”, “C:/path/to/binary/IEDriverServer.exe”);
But it has number of disadvantages to link directly the binary file into our source code as we have to check manually when the new versions of the binaries are released. In addition, our browser version can be updated somehow then we may get compatible issue with the binary file (executable file). To resolved this type of issue, WebDriverManager comes to the picture. It’s an API for Selenium what comes in a JAR format. Means it’s a class library. It allows to automate the management of the binary drivers (e.g. chromedriver, geckodriver, etc.). In order to use WebDriverManager in a Maven project, all we need to add the following dependency in our pom.xml (Java 8 or upper required):
<dependency>
<groupId>io.github.bonigarcia</groupId>
<artifactId>webdrivermanager</artifactId>
<version>3.7.1</version>
<scope>test</scope>
</dependency>
WebDriverManager actually gets our browser version and downloads the compatible browser binary by itself due to run our code without interruption.
Now, rather than setting the browser binaries, we just need to add a single line below and forget about the binary file issues:
WebDriverManager.chromedriver().setup();
driver = new ChromeDriver();
Simply adding a single line as WebDriverManager.chromedriver().setup();
WebDriverManager does magic for us:
- It checks the version of the browser installed in our machine (e.g. Chrome, Firefox).
- It checks the version of the driver (e.g. chromedriver, geckodriver). If unknown, it uses the latest version of the driver.
- It downloads the WebDriver binary if it is not present on the WebDriverManager cache (
~/.m2/repository/webdriver
by default).
More benefits of the WebDriverManagers:
- For each and every browser we can add setup the particular WebDriverManager instance as below:
WebDriverManager.chromedriver().setup();
WebDriverManager.firefoxdriver().setup();
WebDriverManager.operadriver().setup();
WebDriverManager.phantomjs().setup();
WebDriverManager.edgedriver().setup();
WebDriverManager.iedriver().setup();
Watch the video as an example to use WebDriverManager in our code:
2. We can also search for the particular binary version as well in the WebDriverManager as following way to code:
Example | Description |
---|---|
WebDriverManager.chromedriver().version("2.26").setup(); |
Force to use version 2.26 of chromedriver |
WebDriverManager.firefoxdriver().arch32().setup(); |
Force to use the 32-bit version of geckodriver |
WebDriverManager.operadriver().forceCache().setup(); |
Force to use the cache version of operadriver |
Additional methods are exposed by WebDriverManager, namely:
getVersions()
: This method allows to find out the list of of available binary versions for a given browser.getBinaryPath()
: This method allows to find out the path of the latest resolved binary.getDownloadedVersion()
: This method allows to find out the version of the latest resolved binary.clearPreferences()
: This methods allows to remove all Java preferences stored previously by WebDriverManager.clearCache()
: This methods allows to remove all binaries previously downloaded by WebDriverManager.
Source: https://github.com/bonigarcia/webdrivermanager