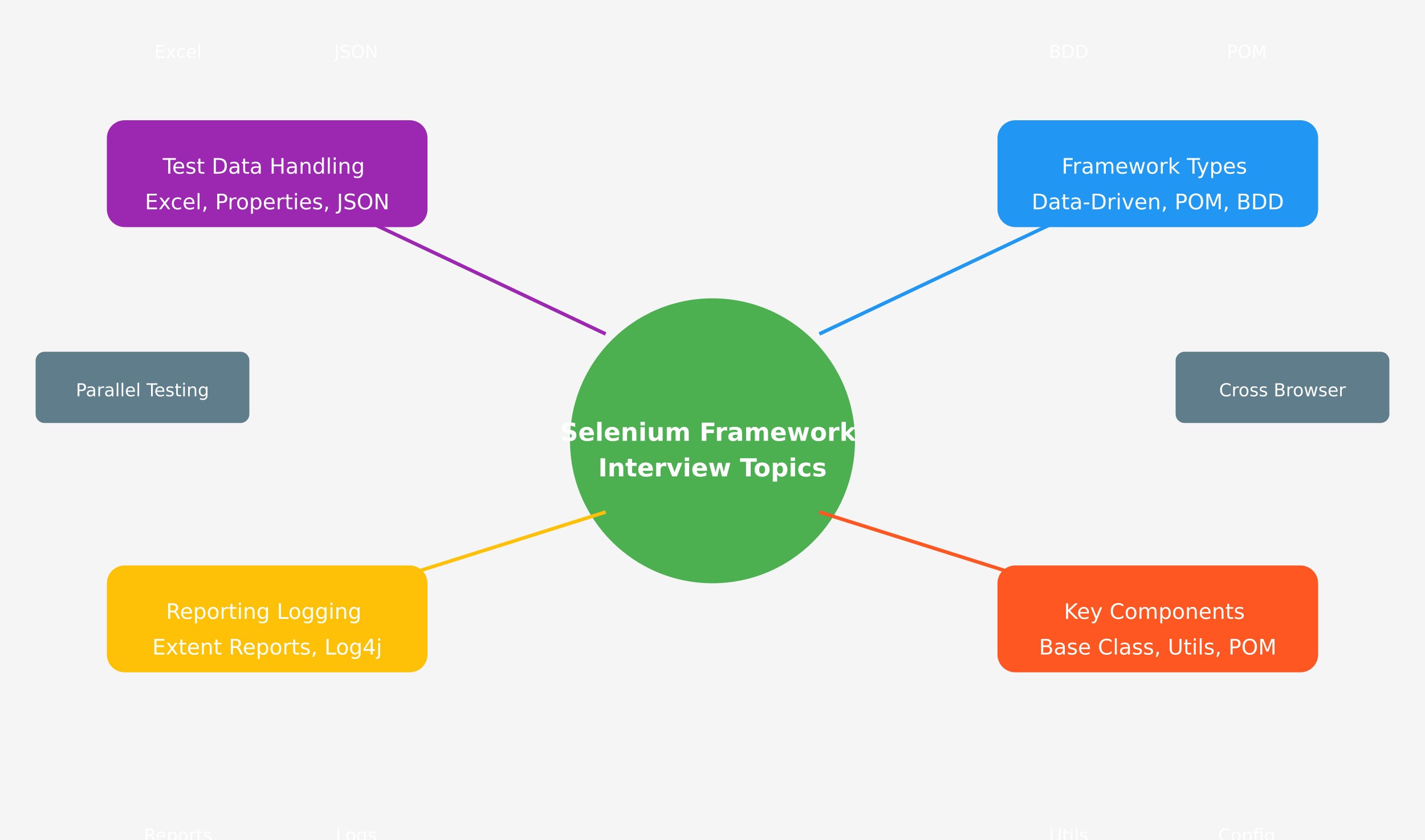
1. What is a Test Automation Framework and why do we need it?
A Test Automation Framework is a set of guidelines, practices, and tools that create a reusable test automation architecture. We need frameworks because they:
- Provide code reusability and maintainability
- Reduce script maintenance costs
- Enhance test efficiency and effectiveness
- Minimize manual intervention
- Generate comprehensive test reports
- Follow coding standards and best practices
- Make the test suite scalable
2. What are the different types of frameworks in Selenium?
There are several types of frameworks commonly used in Selenium:
a) Data-Driven Framework
- Separates test data from test scripts
- Stores test data in external files (Excel, CSV, XML)
- Allows testing with multiple data sets
- Best for scenarios with multiple data combinations
b) Keyword-Driven Framework
- Separates test logic from test scripts
- Uses keywords to represent actions
- Stores keywords in external files
- Non-technical testers can create test cases
c) Hybrid Framework
- Combines multiple framework approaches
- Takes advantages of different frameworks
- Most commonly used in enterprise applications
- Flexible and scalable
d) Page Object Model (POM)
- Creates separate class for each web page
- Encapsulates page elements and methods
- Reduces code duplication
- Improves maintenance
e) Behavior Driven Development (BDD)
- Uses natural language specifications
- Bridges communication gap between technical and non-technical team members
- Popular frameworks: Cucumber, SpecFlow
- Uses Gherkin syntax (Given-When-Then)
3. What are the key components of a good Selenium framework?
A good Selenium framework should include:
- Base Class
- Contains common methods
- Handles driver initialization
- Manages configuration
- Configuration Files
- Store environment variables
- Contains test data
- Manages browser settings
- Utility Classes
- Common reusable methods
- Helper functions
- Generic operations
- Page Objects
- Web element repositories
- Page-specific methods
- Screen interactions
- Test Cases
- Actual test scripts
- Test scenarios
- Assertions
- Reporting Mechanism
- Test execution reports
- Screenshots
- Logging
4. What is Page Object Model (POM) and what are its advantages?
POM is a design pattern that creates an object repository for storing web elements. Each web page is represented as a Java class:
Advantages:
- Reduces code duplication
- Improves test maintenance
- Enhances code reusability
- Makes code more readable
- Separates page objects from test methods
- Provides better error handling
Example Implementation:
public class LoginPage {
private WebDriver driver;
@FindBy(id = "username")
private WebElement usernameField;
@FindBy(id = "password")
private WebElement passwordField;
@FindBy(id = "loginButton")
private WebElement loginButton;
public LoginPage(WebDriver driver) {
this.driver = driver;
PageFactory.initElements(driver, this);
}
public void login(String username, String password) {
usernameField.sendKeys(username);
passwordField.sendKeys(password);
loginButton.click();
}
}
5. How do you handle test data in your framework?
Test data can be handled through multiple approaches:
1. Properties Files
Properties prop = new Properties();
FileInputStream fis = new FileInputStream(“config.properties”);
prop.load(fis);
String url = prop.getProperty(“url”);
2. Excel Files
FileInputStream fis = new FileInputStream(“testdata.xlsx”);
XSSFWorkbook workbook = new XSSFWorkbook(fis);
XSSFSheet sheet = workbook.getSheet(“Login”);
String username = sheet.getRow(1).getCell(0).getStringCellValue();
3. JSON Files
JSONParser parser = new JSONParser();
Object obj = parser.parse(new FileReader(“testdata.json”));
JSONObject jsonObject = (JSONObject) obj;
String username = (String) jsonObject.get(“username”);
6. How do you implement logging in your framework?
Logging is typically implemented using log4j2:
public class TestBase {
public static Logger log = LogManager.getLogger(TestBase.class.getName());
public void someMethod() {
log.info("Starting test execution");
log.error("Test failed with exception");
log.debug("Debugging information");
} }
7. How do you handle reporting in your framework?
Reporting can be implemented using various tools:
- TestNG Reports
- Extent Reports
- Allure Report
8. How do you handle different environments in your framework?
Environment management can be handled through:
1. Properties Files
# qa.properties
url=https://qa.example.com
username=qauser
password=qapass
# prod.properties
url=https://prod.example.com
username=produser
password=prodpass
2. Maven Profiles
<profiles>
<profile>
<id>qa</id>
<properties>
<env>qa</env>
</properties>
</profile>
<profile>
<id>prod</id>
<properties>
<env>prod</env>
</properties>
</profile>
</profiles>
9. How do you handle browser configurations in your framework?
Browser configurations can be managed through:
public class BrowserFactory {
public static WebDriver getDriver(String browserName) {
if(browserName.equalsIgnoreCase("chrome")) {
WebDriverManager.chromedriver().setup();
return new ChromeDriver();
}
else if(browserName.equalsIgnoreCase("firefox")) {
WebDriverManager.firefoxdriver().setup();
return new FirefoxDriver();
}
return null;
}
}
10. How do you handle parallel execution in your framework?
Parallel execution can be implemented using:
- TestNG XML
<suite name="Suite" parallel="methods" thread-count="3">
<test name="Test">
<classes>
<class name="com.test.TestClass"/>
</classes>
</test>
</suite>
2. Grid Configuration
DesiredCapabilities caps = new DesiredCapabilities();
caps.setBrowserName("chrome");
WebDriver driver = new RemoteWebDriver(new URL("http://hub:4444/wd/hub"), caps);
***
π£TRANSFORM your testing skills and learn automation confidently with the best Udemy course: “Master Selenium WebDriver with Java: Scratch to Advance + CI/CD” β a comprehensive guide designed to take you from beginner to expert!π‘ |