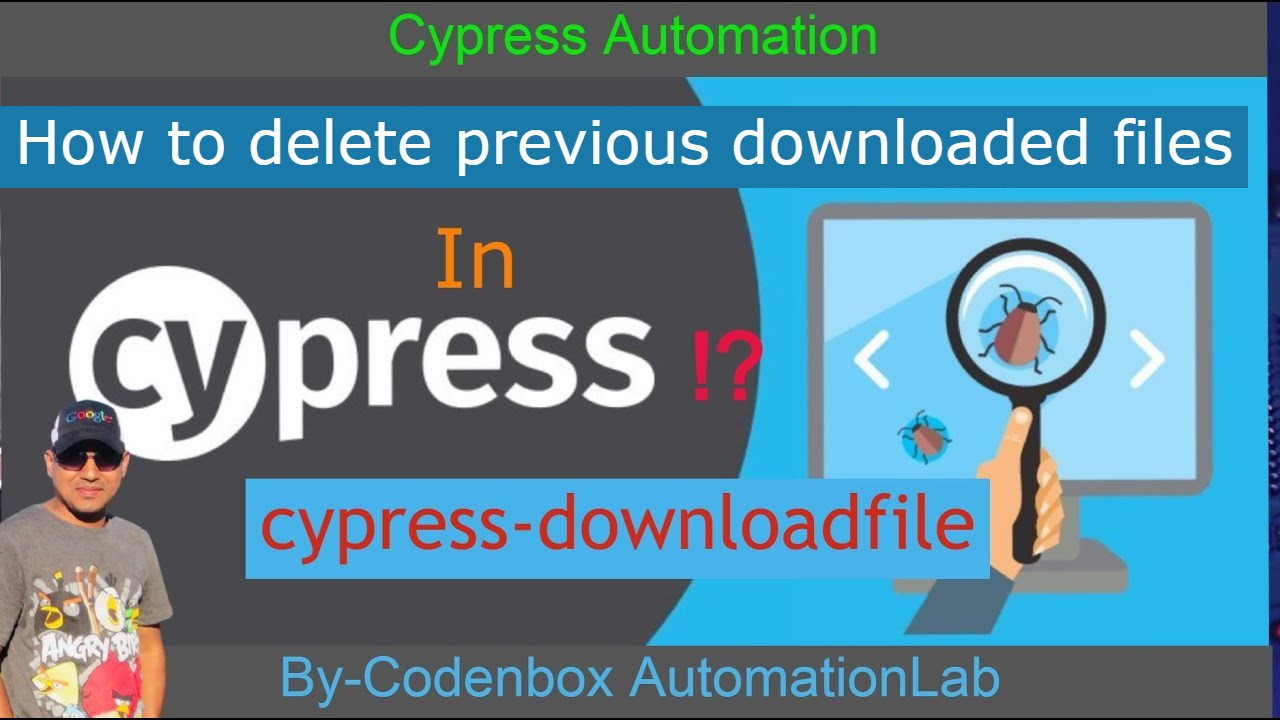
To delete previous downloaded files in Cypress, you can use the fs
(file system) module to delete the files before each test run.
First, you will need to install the fs
module by running the following command in your terminal:
npm install fs
Next, you can use the fs.unlinkSync() function to delete the files before each test run. You can do this by adding the following code to your Cypress support file (e.g. cypress/support/index.js):
import fs from 'fs';
beforeEach(() => {
// Delete all files in the download directory
fs.readdirSync('./downloads').forEach((file) => {
fs.unlinkSync(`./downloads/${file}`);
});
});
This code will delete all files in the downloads directory before each test run.
Alternatively, you can use the fs.rmdirSync() function to delete the entire downloads directory and recreate it before each test run. Here is an example of how you can do this:
import fs from 'fs';
beforeEach(() => {
// Delete the download directory and recreate it
fs.rmdirSync('./downloads', { recursive: true });
fs.mkdirSync('./downloads');
});