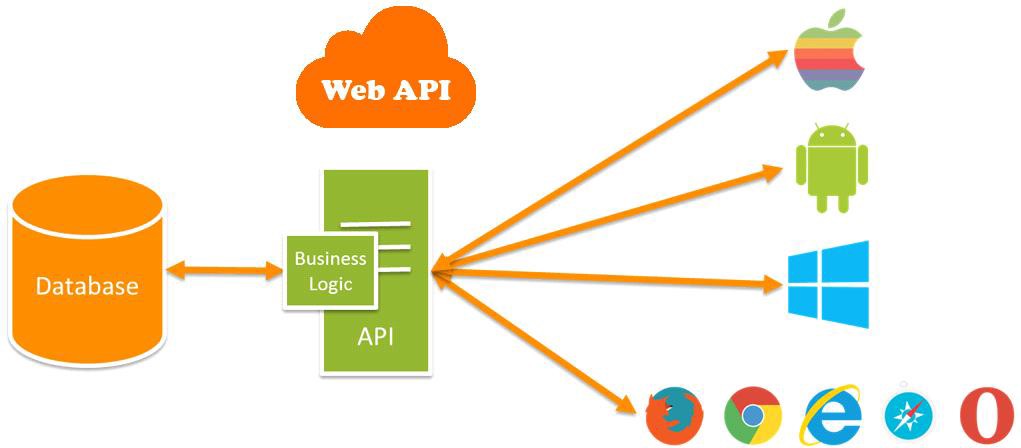
Scripting in Postman
Postman has a powerful runtime based on Node.js that allows you to add dynamic behavior to requests and collections. This allows you to write API tests, build requests that can contain dynamic parameters, pass data between requests, and a lot more. You can add JavaScript code to execute during two events in the flow:
- Before a request is sent to the server, as a pre-request script under the Pre-request Script tab.
- After a response is received, as a test script under the Tests tab.
You can add pre-request and test scripts to a collection, a folder, a request within a collection, or a request not saved to a collection.
- A pre-request script associated with a collection/folder will run prior to every request in the collection.
- A test script associated with a collection/folder will run after every request in that collection/folder.
- A test script associated with a request will run after every time that request will execute.
Getting started with tests:
To write your first test script, open a request in Postman, then select the Tests tab. Write your test script following JavaScript code format:
pm.test(“description ” , function(){
// code for test body
}
Here, pm
refer to Postman library to run the test
method. The text string will appear in the test output. The function inside the test represents an assertion. Postman tests can use Chai Assertion Library BDD syntax.
Let’s have few examples to write Test Script following ‘Dummy API’ :
Testing status codes (GET):
pm.test(“Status code is 200”, function () {
pm.response.to.have.status(200);
pm.expect(pm.response.code).to.eql(200);
pm.expect(pm.response.code).to.be.oneOf([201,202]);
pm.response.to.have.status(“OK”);
});
Testing response body (GET-Single User) :
const responseJson = pm.response.json();
pm.test(“Person is Tiger”, function() {
pm.response.to.have.property(“status”);
pm.expect(responseJson.data.employee_name).to.eql(“Tiger Nixon”);
pm.expect(responseJson.data.employee_age).to.eql(61);
});
Testing header (POST):
pm.test(“check Content-Type header is present”, function()
{pm.response.to.have.header(“Content-Type”);
});
pm.test(“check Content-Type header value”, function()
{
pm.response.to.be.header(“Content-Type”, “application/json”);
or: pm.expect(pm.response.headers.get(‘Content-Type’)).to.eql(‘application/json’);
});
Testing Cookies (PUT):
pm.test(“Cookie ezosuibasgeneris-1 is present”, function() {
pm.expect(pm.cookies.has(‘ezosuibasgeneris-1’)).to.be.true;
});
Test for a particular cookie value:
pm.test("Cookie ezosuibasgeneris-1 has value", function() {
pm.expect(pm.cookies.get('ezosuibasgeneris-1')).to.eql('d4106f44-4c10-4943-6a32-60868d9be5b6');
});
Resource: Learn more..